Design a class that acquires the JSON string from question #1 and converts it to a class data member dictionary. Your class produces data sorted by key or value but not both. Provide searching by key capabilities to your class. Provide string functionality to convert the dictionary back into a JSON string. question #1: import requests from bs4 import BeautifulSoup import json class WebScraping: def __init__(self,url): self.url = url self.response = requests.get(self.url) self.soup = BeautifulSoup(self.response.text, 'html.parser') def extract_data(self): data = [] lines = self.response.text.splitlines()[57:] # Skip the first 57 lines for line in lines: if line.startswith('#'): # Skip comment lines continue values = line.split() row = { 'year': int(values[0]), 'month': int(values[1]), 'decimal_date': float(values[2]), 'average': float(values[3]), 'de_season': float(values[4]), 'days': int(values[5]), 'st_dev': float(values[6]), 'uncertainty': float(values[7]) } data.append(row) # Convert the data list to a JSON string json_data = json.dumps(data) return json_data url = 'https://gml.noaa.gov/webdata/ccgg/trends/co2/co2_mm_mlo.txt' web_scraping = WebScraping(url) json_data = web_scraping.extract_data() print(json_data)
Types of Linked List
A sequence of data elements connected through links is called a linked list (LL). The elements of a linked list are nodes containing data and a reference to the next node in the list. In a linked list, the elements are stored in a non-contiguous manner and the linear order in maintained by means of a pointer associated with each node in the list which is used to point to the subsequent node in the list.
Linked List
When a set of items is organized sequentially, it is termed as list. Linked list is a list whose order is given by links from one item to the next. It contains a link to the structure containing the next item so we can say that it is a completely different way to represent a list. In linked list, each structure of the list is known as node and it consists of two fields (one for containing the item and other one is for containing the next item address).
Design a class that acquires the JSON string from question #1 and converts it to a class data member dictionary. Your class produces data sorted by key or value but not both. Provide searching by key capabilities to your class. Provide string functionality to convert the dictionary back into a JSON string.
question #1:
import requests
from bs4 import BeautifulSoup
import json
class WebScraping:
def __init__(self,url):
self.url = url
self.response = requests.get(self.url)
self.soup = BeautifulSoup(self.response.text, 'html.parser')
def extract_data(self):
data = []
lines = self.response.text.splitlines()[57:] # Skip the first 57 lines
for line in lines:
if line.startswith('#'): # Skip comment lines
continue
values = line.split()
row = {
'year': int(values[0]),
'month': int(values[1]),
'decimal_date': float(values[2]),
'average': float(values[3]),
'de_season': float(values[4]),
'days': int(values[5]),
'st_dev': float(values[6]),
'uncertainty': float(values[7])
}
data.append(row)
# Convert the data list to a JSON string
json_data = json.dumps(data)
return json_data
url = 'https://gml.noaa.gov/webdata/ccgg/trends/co2/co2_mm_mlo.txt'
web_scraping = WebScraping(url)
json_data = web_scraping.extract_data()
print(json_data)

Step by step
Solved in 2 steps

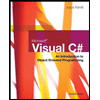
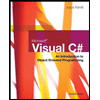