Description Write a program that asks the user for an integer value greater than zero. It will verify that the user has entered a valid value and then try to allocate enough space using malloc for an array of n elements where n is the integer they entered in the first step. Your program should make sure that the allocation worked and then call a function named fillArray that uses a for loop and the square brackets operator to fill the array with the first n odd numbers. For example, the variable that is zero elements away from the base address should get the value 1, the value that is 1 element away from the base address should get the value 3, and so on (see the screen shot below). Afterwards, call another function called printDown that uses another for loop and the square brackets operator to print them in reverse order separated by new lines. Print a line that has 3 asterisks (**") in it by itself. Finally, call another function called printUp that uses another for loop that prints all of the array elements in order but without using the square bracket operator. To do this, you will need to use only the dereference operator and pointer arithmetic (i.e., increment or decrement the pointer). Don't forget to free the memory before the program ends. Your program output should look like the following (please use the same working and format)
Description
Write a program that asks the user for an integer value greater than zero. It will verify that the user has entered a valid value and then try to allocate enough space using malloc for an array of n elements where n is the integer they entered in the first step.
Your program should make sure that the allocation worked and then call a function named fillArray that uses a for loop and the square brackets operator to fill the array with the first n odd numbers. For example, the variable that is zero elements away from the base address should
get the value 1, the value that is 1 element away from the base address should get the value 3, and so on (see the screen shot below). Afterwards, call another function called printDown that uses another for loop and the square brackets operator to print them in reverse order separated by
new lines.
Print a line that has 3 asterisks (''*,,) in it by itself. Finally, call another function called printUp that uses another for loop that prints all of the array elements in order but without using the square bracket operator. To do this, you will need to use only the dereference operator and pointer arithmetic (i.e., increment or decrement the pointer).
Don't forget to free the memory before the program ends.
Your program output should look like the following (please use the same working and format)



Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

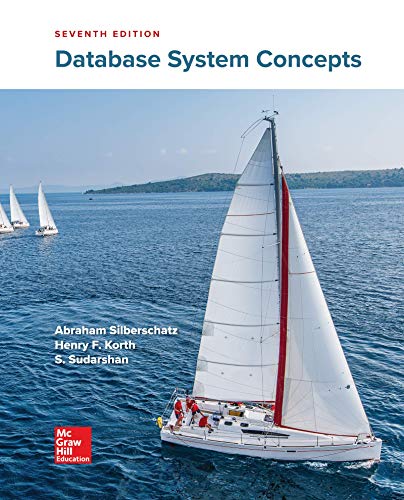
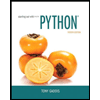
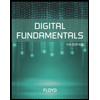
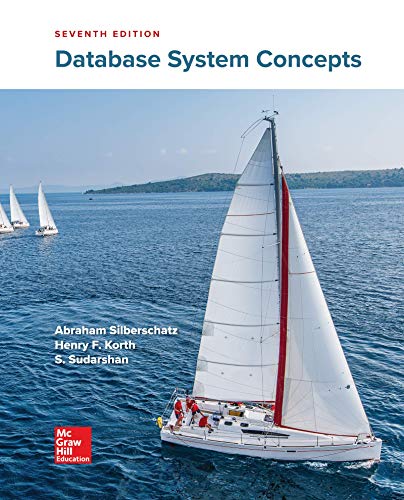
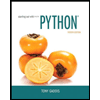
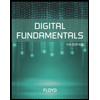
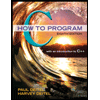
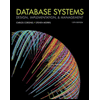
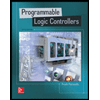