computer graphics simple utility curvei make this code in c#.net but i have error and cant fix it in visual studioi need to fix code and i need to make it to draw curve the design is the picture this is code using System;using System.Drawing;using System.Windows.Forms; public class FunctionCurveForm : Form{ private PictureBox displayArea; private TextBox functionInput; private Button drawButton; private Label functionLabel; public FunctionCurveForm() { InitializeComponent(); } private void InitializeComponent() { this.displayArea = new System.Windows.Forms.PictureBox(); this.functionInput = new System.Windows.Forms.TextBox(); this.drawButton = new System.Windows.Forms.Button(); this.functionLabel = new System.Windows.Forms.Label(); ((System.ComponentModel.ISupportInitialize)(this.displayArea)).BeginInit(); this.SuspendLayout(); // // displayArea // this.displayArea.BackColor = System.Drawing.Color.White; this.displayArea.Location = new System.Drawing.Point(10, 10); this.displayArea.Name = "displayArea"; this.displayArea.Size = new System.Drawing.Size(400, 300); this.displayArea.TabIndex = 0; this.displayArea.TabStop = false; // // functionInput // this.functionInput.Location = new System.Drawing.Point(80, 320); this.functionInput.Name = "functionInput"; this.functionInput.Size = new System.Drawing.Size(230, 22); this.functionInput.TabIndex = 2; // // drawButton // this.drawButton.Location = new System.Drawing.Point(320, 320); this.drawButton.Name = "drawButton"; this.drawButton.Size = new System.Drawing.Size(90, 22); this.drawButton.TabIndex = 3; this.drawButton.Text = "Draw"; // // functionLabel // this.functionLabel.AutoSize = true; this.functionLabel.Location = new System.Drawing.Point(10, 320); this.functionLabel.Name = "functionLabel"; this.functionLabel.Size = new System.Drawing.Size(60, 16); this.functionLabel.TabIndex = 1; this.functionLabel.Text = "Function:"; // // FunctionCurveForm // this.ClientSize = new System.Drawing.Size(430, 380); this.Controls.Add(this.displayArea); this.Controls.Add(this.functionLabel); this.Controls.Add(this.functionInput); this.Controls.Add(this.drawButton); this.Name = "FunctionCurveForm"; this.Text = "Function Curve Drawer"; ((System.ComponentModel.ISupportInitialize)(this.displayArea)).EndInit(); this.ResumeLayout(false); this.PerformLayout(); } private void DrawButton_Click(object sender, EventArgs e) { DrawFunction(); } private void DrawFunction() { using (Graphics g = this.displayArea.CreateGraphics()) { g.Clear(Color.White); Pen pen = new Pen(Color.Black); for (int x = 0; x < this.displayArea.Width; x++) { int y = (int)(100 * Math.Sin(x * 0.05)); // Example function g.DrawRectangle(pen, x, this.displayArea.Height / 2 - y, 1, 1); } } } [STAThread] static void Main() { Application.EnableVisualStyles(); Application.SetCompatibleTextRenderingDefault(false); Application.Run(new FunctionCurveForm()); }}
simple utility curve
i make this code in c#.net but i have error and cant fix it in visual studio
i need to fix code and i need to make it to draw curve
the design is the picture
this is code
using System;
using System.Drawing;
using System.Windows.Forms;
public class FunctionCurveForm : Form
{
private PictureBox displayArea;
private TextBox functionInput;
private Button drawButton;
private Label functionLabel;
public FunctionCurveForm()
{
InitializeComponent();
}
private void InitializeComponent()
{
this.displayArea = new System.Windows.Forms.PictureBox();
this.functionInput = new System.Windows.Forms.TextBox();
this.drawButton = new System.Windows.Forms.Button();
this.functionLabel = new System.Windows.Forms.Label();
((System.ComponentModel.ISupportInitialize)(this.displayArea)).BeginInit();
this.SuspendLayout();
//
// displayArea
//
this.displayArea.BackColor = System.Drawing.Color.White;
this.displayArea.Location = new System.Drawing.Point(10, 10);
this.displayArea.Name = "displayArea";
this.displayArea.Size = new System.Drawing.Size(400, 300);
this.displayArea.TabIndex = 0;
this.displayArea.TabStop = false;
//
// functionInput
//
this.functionInput.Location = new System.Drawing.Point(80, 320);
this.functionInput.Name = "functionInput";
this.functionInput.Size = new System.Drawing.Size(230, 22);
this.functionInput.TabIndex = 2;
//
// drawButton
//
this.drawButton.Location = new System.Drawing.Point(320, 320);
this.drawButton.Name = "drawButton";
this.drawButton.Size = new System.Drawing.Size(90, 22);
this.drawButton.TabIndex = 3;
this.drawButton.Text = "Draw";
//
// functionLabel
//
this.functionLabel.AutoSize = true;
this.functionLabel.Location = new System.Drawing.Point(10, 320);
this.functionLabel.Name = "functionLabel";
this.functionLabel.Size = new System.Drawing.Size(60, 16);
this.functionLabel.TabIndex = 1;
this.functionLabel.Text = "Function:";
//
// FunctionCurveForm
//
this.ClientSize = new System.Drawing.Size(430, 380);
this.Controls.Add(this.displayArea);
this.Controls.Add(this.functionLabel);
this.Controls.Add(this.functionInput);
this.Controls.Add(this.drawButton);
this.Name = "FunctionCurveForm";
this.Text = "Function Curve Drawer";
((System.ComponentModel.ISupportInitialize)(this.displayArea)).EndInit();
this.ResumeLayout(false);
this.PerformLayout();
}
private void DrawButton_Click(object sender, EventArgs e)
{
DrawFunction();
}
private void DrawFunction()
{
using (Graphics g = this.displayArea.CreateGraphics())
{
g.Clear(Color.White);
Pen pen = new Pen(Color.Black);
for (int x = 0; x < this.displayArea.Width; x++)
{
int y = (int)(100 * Math.Sin(x * 0.05)); // Example function
g.DrawRectangle(pen, x, this.displayArea.Height / 2 - y, 1, 1);
}
}
}
[STAThread]
static void Main()
{
Application.EnableVisualStyles();
Application.SetCompatibleTextRenderingDefault(false);
Application.Run(new FunctionCurveForm());
}
}

Step by step
Solved in 2 steps

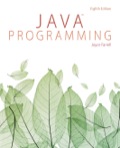
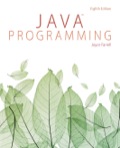