CSC110 Lecture 4_ Defining Functions
.pdf
keyboard_arrow_up
School
University of Toronto *
*We aren’t endorsed by this school
Course
110
Subject
Computer Science
Date
May 17, 2024
Type
Pages
9
Uploaded by CommodoreKnowledgeGoose32 on coursehero.com
CSC110 Lecture 4: Defining Functions
Print this handout
Exercise 1: Reviewing the parts of a function
definition
You are given the following function definition of a Python function.
Answer the following questions about this definition.
1. What is the function name
in this definition?
2. What is the function header
in this definition?
3. How many parameters
does this function have? What is the name
and type
of each parameter?
4. What is the function’s return type
?
def
calculate(x: int, y: int) -> list:
"""Return a list containing the sum and product of the two given numbers.
"""
return
[x + y, x * y]
5. What is the part surrounded by triple-quotes (
"""
) called? What is its purpose?
6. What is the function body
in this definition?
7. Compared to the example function definitions we looked at in lecture, what part is missing from this
function definition?
8. Write down what you would add to complete this function definition.
Exercise 2: The Function Design Recipe
For your reference, here are the steps of the Function Design Recipe
:
1. Write examples uses
2. Write the function header
3. Write the function description
4. Implement the function body
5. Test the function
1. Consider the following problem description:
Given two lists, return whether they have the same length.
We have started the Function Design Recipe for you. Complete the code below to solve this problem by
following the steps of the Function Design Recipe.
2. Now consider this problem description:
Given a float
representing the price of a product and another float
representing a tax rate
(e.g., a 10% tax rate represented as the float
value 0.1
), calculate the after-tax cost of the
product, rounded to two decimal places.
Again, complete the code below to solve this problem by following the steps of the Function Design
Recipe.
3. Finally, consider this problem description:
Given a list of product prices (as float
s) and a tax rate, calculate the total
after-tax cost of
all the products in the list rounded to two decimal places.
To keep things simple, don’t worry about any rounding errors in your calculation.
def
is_same_length(list1: list, list2: list) -> bool:
"""
>>> is_same_length([1, 2, 3], [4, 5, 6])
True
>>> is_same_length([1, 2, 3], [])
False
"""
"""
>>> after_tax_cost(5.0, 0.01)
5.05
"""
This time, we haven’t provided you any code—follow the Function Design Recipe to write a complete
function that solves this problem.
Tip
: to keep your doctest examples numerically simple, try using a tax rate of 0.5
or 1.0
.
Exercise 3: Practice with methods
Note
: you might want to use Appendix A.2 Python Built-In Data Types Reference
(https://www.teach.cs.toronto.edu/~csc110y/fall/notes/A-python-builtins/02-types.html) as a reference in
this exercise.
1. Suppose we have executed the following assignment statements in the Python console:
Write down what each of the following expressions evaluate to.
Do this by hand first! (Then check your work in the Python console. Don’t worry if you wrote set
elements in a different order that what appears in the Python console.)
>>> wish = 'Happy Birthday'
>>> set1 = {
1
, 2
, 3
}
>>> set2 = {
2
, 4
, 5
, 10
}
>>> strings = [
'cat'
, 'rabbit'
, 'dog'
, 'rabbit'
]
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
What is the difference between call by reference and call by value when it comes to
Memory usage
data types of the function parameters
arrow_forward
What roles do the parameters and the return statement play in a function definition?
arrow_forward
• Why do we use function prototypes?
• What is the difference between arguments and parameters?
• When to use local variables and when to use parameters?
arrow_forward
Explain the purpose of a function parameter. What’s the difference between a parameterand an argument?
arrow_forward
What is the difference between a formal parameter and an argument?
Group of answer choices
A function’s argument is referred to as the formal argument to distinguish it from the value that is passed in during the function call.
The parameter is the passed value.
A function’s parameter is referred to as the formal parameter to distinguish it from the value that is passed in during the function call.
The argument is the passed value.
A function’s parameter is used for passing by reference.
The argument is used for passing by value.
A function’s parameter is used for passing by value.
The argument is used for passing by reference.
They are identical but using different terms.
arrow_forward
. What is the difference between a function argument and a function parameter?
Group of answer choices
An argument is a variable that is created in a function to hold a value that has been sent to it and a parameter value is the value that a function returns to the calling routine.
An argument is a value that is passed into a function and a parameter is variable that the function defines to hold value of the argument.
An argument is a value that is passed into a function and a parameter is the value that a function returns to the calling routine.
A parameter is a value that is passed into a function and an argument is the value that a function returns to the calling routine.
arrow_forward
The scope of a function contains..
a. all variables defined within the function and the function parameters.
b. all variables defined within the function but not the function parameters
c. all variables in the program no matter where they are defined.
d. only the function parameters
arrow_forward
Define the terms function header, parameter, and argument.
arrow_forward
What is the definition of a function?
arrow_forward
PROGRAMMING LANGUAGE: C++
Note: Kindly refer to the attached screenshot for the sample program.
1. Identify the format of the function used: no return type & no parameter, with return type & no parameter, no return type & with parameter and with return type & with parameter.
2. Identify the format and actual parameters.
3. Identify the function signature
4. Identify parameter if value parameter, reference parameter or constant reference parameter is used.
5. Identify the the scope of variable used.
6. Identify the type of C++ function is used.
arrow_forward
void fx(char
ch) is a
function with
no return
value
int fx(char x)
is a function
with int
return value
int fx(char
&x) is a
function with
parameter
passed by
value
char fx( int x)
is a function
with int
return value
arrow_forward
The Issue: You have a function that accepts a variety of parameters. It is impractical to provide them as traditional parameters. So, what are you going to do?
arrow_forward
A function has different forms such as function call ,declaration, definition .in your opinion
which form of function is called prototype and why?
arrow_forward
True or False : The concept of function abstraction hinders our code development by confusing us with the details of the function.
arrow_forward
3- Write a python function that takes in one integer as a parameter. It makes sure that the number is indeed an integer and prints the sum of all its digits. Sample input: 145
Sample output: 1+4+5=10
arrow_forward
write a function that prints your name and ID.
include a function definition, declaration, and function call
arrow_forward
Using C++ Programming language:
Assume you want a function which expects as parameters an array of doubles and the size of the array. Write the function header that accepts these parameters but is defined in such a way that the array cannot be modified in the function. You can use your own variable names for the parameters.
arrow_forward
C++ The function declaration part of this program is missing. Your job is to insert the function prototypes for all user-defined functions into the program. First, decide how many and what are the user-defined functions (exclude the main function) in this program. Then, declare the function prototype of each function in the appropriate place in the program.
arrow_forward
Parameters are the value passed to a function when the function is called and Argument are the variable defined in the function definition.
True or false
arrow_forward
Convert radians into degrees
Write a function in Python that accepts one numeric parameter. This
parameter will be the measure of an angle in radians. The function should
convert the radians into degrees and then return that value.
arrow_forward
True or False
1-int fx(char x) is a function with int return
value
2-int fx(char &x) is a function with parameter
passed by value
3-void fx(char ch) is a function with no return
value
4-char fx( int x) is a function with int return
value
arrow_forward
What are the benefits of using a function?
arrow_forward
What is the return type of a main function?
arrow_forward
1-int fx(char &x) is a function with parameter passed by value(False/True)
2-int fx(char x) is a function with int return value(False/True)
3-void fx(char ch) is a function with no return value(False/True)
4-char fx( int x) is a function with int return value(False/True)
arrow_forward
What is Function Overloading? How we can implement more than one function in the program with the same function name?
arrow_forward
13.
What is the difference between a formal parameter and an argument?
Group of answer choices
A function’s argument is referred to as the formal argument to distinguish it from the value that is passed in during the function call.
The parameter is the passed value.
They are identical but using different terms.
A function’s parameter is used for passing by reference.
The argument is used for passing by value.
A function’s parameter is referred to as the formal parameter to distinguish it from the value that is passed in during the function call.
The argument is the passed value.
A function’s parameter is used for passing by value.
The argument is used for passing by reference.
arrow_forward
write python code for functions:
arrow_forward
Local declarations are those that are kept in the memory of the computer; but, how exactly are they kept in memory? If reaching one's objective can be done without making use of local declarations, then doing so is a waste of time. Why bother using value parameters when you can just use references as your arguments in any function? How crucial are value parameters when it comes to the processing of programmed data?
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
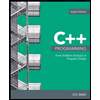
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Related Questions
- What is the difference between call by reference and call by value when it comes to Memory usage data types of the function parametersarrow_forwardWhat roles do the parameters and the return statement play in a function definition?arrow_forward• Why do we use function prototypes? • What is the difference between arguments and parameters? • When to use local variables and when to use parameters?arrow_forward
- Explain the purpose of a function parameter. What’s the difference between a parameterand an argument?arrow_forwardWhat is the difference between a formal parameter and an argument? Group of answer choices A function’s argument is referred to as the formal argument to distinguish it from the value that is passed in during the function call. The parameter is the passed value. A function’s parameter is referred to as the formal parameter to distinguish it from the value that is passed in during the function call. The argument is the passed value. A function’s parameter is used for passing by reference. The argument is used for passing by value. A function’s parameter is used for passing by value. The argument is used for passing by reference. They are identical but using different terms.arrow_forward. What is the difference between a function argument and a function parameter? Group of answer choices An argument is a variable that is created in a function to hold a value that has been sent to it and a parameter value is the value that a function returns to the calling routine. An argument is a value that is passed into a function and a parameter is variable that the function defines to hold value of the argument. An argument is a value that is passed into a function and a parameter is the value that a function returns to the calling routine. A parameter is a value that is passed into a function and an argument is the value that a function returns to the calling routine.arrow_forward
- The scope of a function contains.. a. all variables defined within the function and the function parameters. b. all variables defined within the function but not the function parameters c. all variables in the program no matter where they are defined. d. only the function parametersarrow_forwardDefine the terms function header, parameter, and argument.arrow_forwardWhat is the definition of a function?arrow_forward
- PROGRAMMING LANGUAGE: C++ Note: Kindly refer to the attached screenshot for the sample program. 1. Identify the format of the function used: no return type & no parameter, with return type & no parameter, no return type & with parameter and with return type & with parameter. 2. Identify the format and actual parameters. 3. Identify the function signature 4. Identify parameter if value parameter, reference parameter or constant reference parameter is used. 5. Identify the the scope of variable used. 6. Identify the type of C++ function is used.arrow_forwardvoid fx(char ch) is a function with no return value int fx(char x) is a function with int return value int fx(char &x) is a function with parameter passed by value char fx( int x) is a function with int return valuearrow_forwardThe Issue: You have a function that accepts a variety of parameters. It is impractical to provide them as traditional parameters. So, what are you going to do?arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
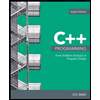
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning