midterm exam2 - sample questions (1)
.pdf
keyboard_arrow_up
School
Miami University *
*We aren’t endorsed by this school
Course
271
Subject
Computer Science
Date
May 13, 2024
Type
Pages
7
Uploaded by DeaconKoala4263 on coursehero.com
These are meant to give you an example of some of the kinds of questions you might be asked on a
midterm. Your exam may or may not have any of these questions on it. Your exam will definitely
include other kinds of questions not found on this sample. Don't study for these particular questions.
But this should give you an idea of the
kinds
of code you will be expected to write and read, concepts
you should understand, and so on. I will not provide answers or check your work on these if you
decide to practice them.
1.
To over
ride
a superclass method in a subclass, the subclass method ____.
a)
Must use the same method name and the same parameter types.
b)
Must include a call to the super class method with the same name.
c)
Must use the same method name and different parameter types.
d)
Must use a different method name.
e)
None of the above
2.
Consider the following code snippet:
public class Motorcycle extends Vehicle {
private String model;
public Motorcycle(int numberAxles, String modelName) {
super(numberAxles);
model = modelName;
}
}
What does the above code do?
a)
It calls a
private
method of the
Vehicle
class from within a method of the
Motorcycle
class.
b)
It calls a
public
method of the
Vehicle
class from within a method of the
Motorcycle
class.
c)
It calls the constructor of the
Vehicle
class from within the constructor of the
Motorcycle
class.
d)
It calls the constructor of the
Motorcycle
class from within the constructor of the
Vehicle
class.
3.
Suppose the
Auto
class extends the
Vehicle
class, and both classes have an implementation of the
public void moveForward(int)
method. Now, in a separate tester class, you write the following:
Vehicle myAuto = new Auto();
Which one of the following would allow you to call the moveForward() method in the Vehicle class?
a) ((Vehicle)myAuto).moveForward(20);
b) ((super)myAuto).moveForward(20);
c) myAuto.super.moveForward(20);
d)
It is not possible to call the
Vehicle
moveForward
method using the
myAuto
variable.
Page
1
of
7
4.
Which of the following will return a negative result?
a)
"aaazbb".compareTo("aaabbb");
b)
"wxyz".compareTo("wx");
c) "abc".compareTo("abcde");
d)
"efg".compareTo("def");
e)
None of the above
Suppose you have the following classes and interfaces, and that all classes have a no-parameter constructor
defined.
public interface Lyftable
public class Vehicle
public abstract class LandVehicle extends Vehicle
Which of the following are valid?
Circle
Yes
or
No
Statement
Valid? (circle yes or no)
LandVehicle v3 = new LandVehicle();
YES
NO
Lyftable c1 = new Cab();
YES
NO
Suppose that a
Media
class has several subclasses. One of the subclasses is
Book
. The
Media
class has a
getTitle()
method that returns the title as a
String
. None of the subclasses has its own
getTitle()
method defined. One of its subclasses,
Book
, has a
getPages()
method that returns the number of pages in
the book as an
int
. The
getPages()
method only exists in the
Book
class, and not in the
Media
class
and not in any of the other subclasses. Write a method named
process()
that has as its only parameter a
Media
array. The array may contain some
null
elements. The method should:
●
Print the title of each non-
null
element in the array.
●
Return the sum of all the pages of all the book objects that are in the array.
●
Contain exactly one loop, and the loop should be a "for each" style loop.
public int process(Media[] media) {
}
Page
2
of
7
Consider the definition for a
Car
. Complete/modify the definition for the
FourWheelDriveCar
class. A
four wheel drive car is to be a child of the
Car
class.
FourWheelDriveCar
cars can be configured with
heavy duty tires (or not).
FourWheelDriveCar
cars are 20% more costly to drive than a normal car if they
are using regular tires and 25% more if they are using heavy duty tires. You should make changes to the
Car
class only if absolutely needed.
Reuse existing code as much as possible.
public class Car {
private String make;
private double mpg;
public Car(String make, double mpg)
{ // code hidden from view }
// Copy constructor
public Car(Car c)
{ // code hidden from view }
// returns the number of gallons needed to travel a specific distance
public double gallonsForTrip(double dist)
{ return dist / mpg; }
public double costOfTrip(double dist)
{
double wearAndTear = dist * 0.25;
double gasCost = 2.45 * gallonsForTrip(dist);
return gasCost + wearAndTear;
}
public String toString()
{ return make + " MPG: " + mpg; }
public String getMake()
{ return make; }
public double getMPG()
{ return mpg; }
public boolean canGoToMaunaKea()
{ return false; }
}
// This is a subclass of Car
public class FourWheelDriveCar
{
private boolean heavyDutyTires;
public FourWheelDriveCar(boolean heavyTires, String make, double mpg){
}
//
CONTINUED ON NEXT PAGE…
Page
3
of
7
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
SET 1 a) Discuss and describe an important idea or hypothesis from the course. b) How can you use this explanation to explain a personal experience? Please be as specific as possible.
The aim of this written submission is for you to focus on the events of your life (work/school/family/friends) and use them as an excuse to put what you've learned in class into reality. Try to answer the following questions when you write your submissions.
1) What did you take away from the encounter?
arrow_forward
Why do you want to go to college? That is the main question for your Written Assignment. You will write one paragraph (three or four sentences) on this topic.
arrow_forward
Tell me about a time when it was hard for you to make your point in an online class or program. Do you have any suggestions for how we could get past these problems?
arrow_forward
SET 1 a) Discuss and describe an important idea or hypothesis from the course. b) How can you use this explanation to explain a personal experience? Please be as specific as possible.
The aim of this written submission is for you to focus on the events of your life (work/school/family/friends) and use them as an excuse to put what you've learned in class into reality. Try to answer the following questions when you write your submissions
3) In your view, what elements of the idea, hypothesis, or suggestion fit well?
arrow_forward
There have been several actors who have played the titular character in the British sci-fi series Doctor Who since the show began in the early 1960s. In 2005 the series was rebooted and Christopher Eccleston, David Tennant, Matt Smith, Peter Capaldi, and Jodie Whittaker have all played the Doctor since. Several Whovians (fans of the show) were asked to rank the actors of the Doctor from their favorite to their least favorite. The results are in the preference schedule below using the initials of each actor (so CE = Christopher Eccleston; DT = David Tennant; etc.).
6
8
12
16
17
18
23
20
1st Choice
CE
DT
DT
DT
MS
MS
PC
JW
2nd Choice
MS
CE
PC
PC
JW
CE
JW
CE
3rd Choice
DT
JW
CE
JW
CE
DT
DT
PC
4th Choice
PC
MS
JW
MS
PC
PC
CE
DT
5th Choice
JW
PC
MS
CE
DT
JW
MS
MS
Is there a Condorcet winner?Answer: Explain:
Do any of the voting methodsPlurality Method, Instant…
arrow_forward
There have been several actors who have played the titular character in the British sci-fi series Doctor Who
since the show began in the early 1960s. In 2005 the series was rebooted and Christopher Eccleston, David
Tennant, Matt Smith, Peter Capaldi, and Jodie Whittaker have all played the Doctor since. Several Whovians
(fans of the show) were asked to rank the actors of the Doctor from their favorite to their least favorite. The
results are in the preference schedule below using the initials of each actor (so CE = Christopher Eccleston;
DT = David Tennant; etc.).
6.
8
12
16
17
18
23
20
1st Choice
DT
DT
DT
MS
MS
PC
JW
CE
2nd Choice
CE
PC
PC
JW
CE
JW
CE
MS
3rd Choice
JW
СЕ
JW
CE
DT
DT
PC
DT
4th Choice
PC
MS
JW
MS
PC
PC
DT
PC
MS
CE
DT
JW
MS
MS
5th
Choice
JW
a. Is there a Condorcet winner?
Answer:
Explain:
b. Do any of the voting methods Plurality Method, Instant Runoff Voting Method, Borda Count Method,
Copeland's Method violate the Majority Criterion?
Answer:
Explain:
arrow_forward
answer the question task 3
question 1, 2 and 3…
arrow_forward
1
Print
Evaluating a Procedural Text
In this task, you'll read a procedural text and then evaluate its organization and clarity, applying the guidelines you read previously. Then you'll suggest ways to improve it. This task will help you become familiar with the components of this type of text before you write your own. Read this written lab procedure, and then answer the questions that follow:
Performing a Lab Experiment
First, you'll need to make sure you have all your equipment, such as a science notebook, dissection tools, a stopwatch, an electronic balance, glassware, and possibly many others. The next thing that you'll want to do is have all your safety equipment—helmet, mask, goggles, ear protection, respirator, welding mask, whatever you need. Always follow these guidelines for safety:
Read the entire lab procedure.
Bring only needed materials into area.
Know where the safety and emergency equipment is located.
It's important to understand how to dispose of waste after…
arrow_forward
Create an analysis connecting one or more points in this article to at least two of the CSE activities you completed earlier this semester. “Connecting” can take a wide variety of forms. For example, you might compare the ideas in this article with the ideas in one of the earlier CSE articles we worked with. You might take a theoretical framework and/or practical case we worked with earlier and apply ideas in this article to the theory, the practice, or both.
(Please type answer no write by hand)
arrow_forward
E3
In this project, you are asked to independently research and write a short report relating to our discussion on Biofuel Energy. Your topic selection is completely of your choosing, though the report must clearly demonstrate how the subject is relevant to the overall topic of Biofuels. This course presents introductory discussions through module content and our weekly lectures, to enhance this learning it is up to the student to further explore and independently research topics of interest. The goal of this research report is to explore what interests you, and get on paper your ideas about this area of renewables technology.
The marking scheme rewards originality and uniqueness of topic selection. Students with same/similar topics will loose significant marks. Plagiarism will result in a 0 mark, and will be dealt with according to Conestoga Academic Integrity policies.
As this is a research report, it is important to develop an overview or a thesis statement about the topic you wish…
arrow_forward
Useful information, but this is not answering the fill-in-the-blank question. Can you help me answer?
arrow_forward
What motivates you to get a degree at a university? In your Written Assignment, you will be asked to answer this question in depth. In response to this subject, you will compose a paragraph (three or four phrases).
arrow_forward
Need help with Question #2.
Question #1 has been provided to you for sample reference along with the work.
arrow_forward
Please help out this practice question (it is not an exam question)
arrow_forward
A company has a large number of documents that need to be sorted into one of three categories: Research & Development, Finance or Marketing. They have been able to identify a number of phrases which are commonly used in these documents and may help disambiguate them but there are a large number (thousands) of these phrases and each one only appears in a small number of documents. Thankfully someone has labeled a few hundred documents for you but you need to design an algorithm to automatically label the rest.
arrow_forward
What picture do you have in your head when you think about e-mail? When a message is sent, how does it get to the person who is supposed to receive it? Make a note of all of the information you've gathered thus far. What are the differences, and why are they important to understand? Consider the degree of detail (or the level of abstraction) that various types of models possess in comparison to one another.
arrow_forward
If you're in charge of choosing and installing an EHR system, you'll need to make arrangements for interviews to determine which candidates will be considered. Choose the best option and purchase it. Make sure you choose the right program by coming up with 5 questions to ask both before and after an interview. Justify your reasoning for each inclusion on the list.
arrow_forward
Why do you want to attend a university to get a degree? You will be required to provide a
thorough response to this question in your written assignment. You are going to write a
paragraph in response to this topic (three or four phrases).
arrow_forward
Correct and detailed answer will be Upvoted. Thank you
arrow_forward
What strategies do you have in place for collecting and interpreting test results?
arrow_forward
What kind of mental picture do you conjure up whenever you think about email? Is it possible for a message to be sent to the individual who it is meant for? It is important to keep track of everything you've studied up to this point. To what degree does one's comprehension of the differences between the two need to be prioritised? How specific (or general) are the various models, and how do they stack up against one another?
arrow_forward
Objective
The objective of this lab is to create the beginnings for a python-based card game. We DO NOT expect a fully functioning card game. What we do expect is that you
create a main function and various functions that will accomplish the following goals:
• Build a single-dimension array to keep track of the location of every card
• DO NOT move cards around.. Just use the array to keep track of where each card is
• All card data is really integers - Use other arrays to translate integers to suits, ranks, and player names
• All cards will start in the DECK
• Write a function that translates a card number to a card name. HINT - look at the suitName and rankName arrays
• Write a function to assign a card to a given player
• Dealing a card involves picking a card number and assigning a new location to the corresponding element of cardLoc
• Write a function that displays the location of every card. (Early versions should show numeric values for the card number and location. Later…
arrow_forward
This is not a graded question so please don't disregard it as if it is.Thank you in advance professor!
Note: This is a theoretical question and should be answered without coding
arrow_forward
What visuals come to mind when you think about email? Where does a message go once you send it through email? Don't forget to jot down all you've learned thus far. What may be the causes of these differences, if anybody knows? Do you accommodate varying degrees of complexity in your models?
arrow_forward
task 3
its related to question 1,2 and 3
just answer question 4 and 5 only…
arrow_forward
This is everything that the question provides. There isn't anything else I can add to make the question more clear
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
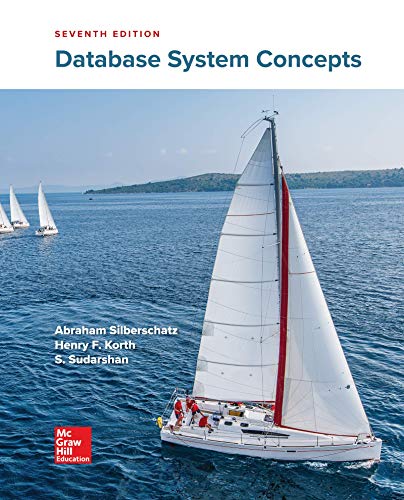
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
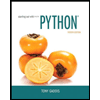
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
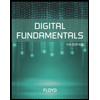
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
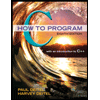
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
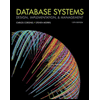
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
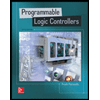
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
Related Questions
- SET 1 a) Discuss and describe an important idea or hypothesis from the course. b) How can you use this explanation to explain a personal experience? Please be as specific as possible. The aim of this written submission is for you to focus on the events of your life (work/school/family/friends) and use them as an excuse to put what you've learned in class into reality. Try to answer the following questions when you write your submissions. 1) What did you take away from the encounter?arrow_forwardWhy do you want to go to college? That is the main question for your Written Assignment. You will write one paragraph (three or four sentences) on this topic.arrow_forwardTell me about a time when it was hard for you to make your point in an online class or program. Do you have any suggestions for how we could get past these problems?arrow_forward
- SET 1 a) Discuss and describe an important idea or hypothesis from the course. b) How can you use this explanation to explain a personal experience? Please be as specific as possible. The aim of this written submission is for you to focus on the events of your life (work/school/family/friends) and use them as an excuse to put what you've learned in class into reality. Try to answer the following questions when you write your submissions 3) In your view, what elements of the idea, hypothesis, or suggestion fit well?arrow_forwardThere have been several actors who have played the titular character in the British sci-fi series Doctor Who since the show began in the early 1960s. In 2005 the series was rebooted and Christopher Eccleston, David Tennant, Matt Smith, Peter Capaldi, and Jodie Whittaker have all played the Doctor since. Several Whovians (fans of the show) were asked to rank the actors of the Doctor from their favorite to their least favorite. The results are in the preference schedule below using the initials of each actor (so CE = Christopher Eccleston; DT = David Tennant; etc.). 6 8 12 16 17 18 23 20 1st Choice CE DT DT DT MS MS PC JW 2nd Choice MS CE PC PC JW CE JW CE 3rd Choice DT JW CE JW CE DT DT PC 4th Choice PC MS JW MS PC PC CE DT 5th Choice JW PC MS CE DT JW MS MS Is there a Condorcet winner?Answer: Explain: Do any of the voting methodsPlurality Method, Instant…arrow_forwardThere have been several actors who have played the titular character in the British sci-fi series Doctor Who since the show began in the early 1960s. In 2005 the series was rebooted and Christopher Eccleston, David Tennant, Matt Smith, Peter Capaldi, and Jodie Whittaker have all played the Doctor since. Several Whovians (fans of the show) were asked to rank the actors of the Doctor from their favorite to their least favorite. The results are in the preference schedule below using the initials of each actor (so CE = Christopher Eccleston; DT = David Tennant; etc.). 6. 8 12 16 17 18 23 20 1st Choice DT DT DT MS MS PC JW CE 2nd Choice CE PC PC JW CE JW CE MS 3rd Choice JW СЕ JW CE DT DT PC DT 4th Choice PC MS JW MS PC PC DT PC MS CE DT JW MS MS 5th Choice JW a. Is there a Condorcet winner? Answer: Explain: b. Do any of the voting methods Plurality Method, Instant Runoff Voting Method, Borda Count Method, Copeland's Method violate the Majority Criterion? Answer: Explain:arrow_forward
- answer the question task 3 question 1, 2 and 3…arrow_forward1 Print Evaluating a Procedural Text In this task, you'll read a procedural text and then evaluate its organization and clarity, applying the guidelines you read previously. Then you'll suggest ways to improve it. This task will help you become familiar with the components of this type of text before you write your own. Read this written lab procedure, and then answer the questions that follow: Performing a Lab Experiment First, you'll need to make sure you have all your equipment, such as a science notebook, dissection tools, a stopwatch, an electronic balance, glassware, and possibly many others. The next thing that you'll want to do is have all your safety equipment—helmet, mask, goggles, ear protection, respirator, welding mask, whatever you need. Always follow these guidelines for safety: Read the entire lab procedure. Bring only needed materials into area. Know where the safety and emergency equipment is located. It's important to understand how to dispose of waste after…arrow_forwardCreate an analysis connecting one or more points in this article to at least two of the CSE activities you completed earlier this semester. “Connecting” can take a wide variety of forms. For example, you might compare the ideas in this article with the ideas in one of the earlier CSE articles we worked with. You might take a theoretical framework and/or practical case we worked with earlier and apply ideas in this article to the theory, the practice, or both. (Please type answer no write by hand)arrow_forward
- E3 In this project, you are asked to independently research and write a short report relating to our discussion on Biofuel Energy. Your topic selection is completely of your choosing, though the report must clearly demonstrate how the subject is relevant to the overall topic of Biofuels. This course presents introductory discussions through module content and our weekly lectures, to enhance this learning it is up to the student to further explore and independently research topics of interest. The goal of this research report is to explore what interests you, and get on paper your ideas about this area of renewables technology. The marking scheme rewards originality and uniqueness of topic selection. Students with same/similar topics will loose significant marks. Plagiarism will result in a 0 mark, and will be dealt with according to Conestoga Academic Integrity policies. As this is a research report, it is important to develop an overview or a thesis statement about the topic you wish…arrow_forwardUseful information, but this is not answering the fill-in-the-blank question. Can you help me answer?arrow_forwardWhat motivates you to get a degree at a university? In your Written Assignment, you will be asked to answer this question in depth. In response to this subject, you will compose a paragraph (three or four phrases).arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
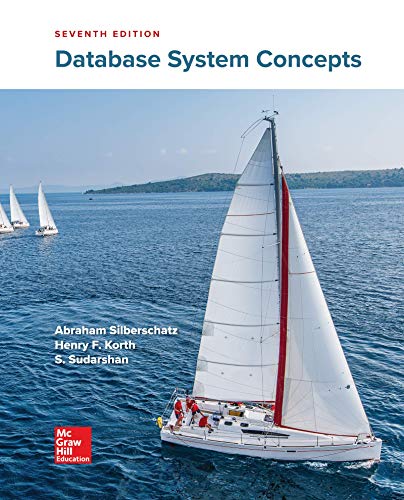
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
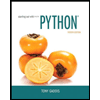
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
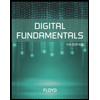
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
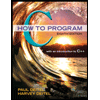
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
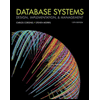
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
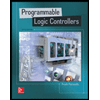
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education