ICT159 - Assignment 2(1)
.docx
keyboard_arrow_up
School
Murdoch University *
*We aren’t endorsed by this school
Course
159
Subject
Computer Science
Date
May 13, 2024
Type
docx
Pages
25
Uploaded by HighnessIbex4352 on coursehero.com
ICT159 Foundations of Programming
ICT159 – Assignment 2
This is an individual assignment, and is worth 20% of the total assessment for the unit.
Student ID
Name
Final Mark
Objectives
Construct algorithms to solve problems using a combination of sequence, selection and iteration constructs.
Implement such algorithms in a common programming language, C
.
Read/write data to/from files.
Use arrays of records (structs).
Search arrays of records.
Apply the methodology of top-down design to the construction of solutions and implement these solutions in a modular way.
Prerequisites
This Assignment extends Assignment 1. There is meant to be code reuse from Assignment 1 if your design and code from Assignment 1 adheres to the principles of high cohesion and low coupling.
You will know if these principles were adhered to if you are able to reuse most of the modules from Assignment 1. Before attempting this question, please complete the following prerequisites:
Make sure you have attempted Assignment 1, even if not submitted.
All lab exercises for modular programming, arrays, Files and Data Structures have been completed.
Make sure that you have corrected your solution to Assignment 1 following the solution discussed during the lectures (please refer to the recorded videos).
This is an individual assignment and must be completed by you alone.
Any unauthorised collaboration/collusion may be subject to investigation
and result in penalties if found to be in breach of University policy.
Submission Guidelines
Your assignment must be submitted via LMS following the guidelines listed below. Failure to adhere to these guidelines will result in marks being lost or a fail grade being awarded.
Create a folder named ICT159_StudentID_Surname_Assignment2. Make sure you replace StudentID with your student ID and Surname with your surname
Put the source code (i.e., C program) files into this folder.
Answer the questions directly on this World document (the C programs need to be included separately; see the previous points. Do not paste code into this word document). Once done, convert it into PDF and save it inside the folder ICT159_StudentID_Surname_Assignment2.
You should always keep a copy of all your work. Keep the backup of your work in cloud storage like OneDrive. Losing your work/files (for any reason) a few days before the deadline is not a valid ground for extension.
Note that:
You may be required to demonstrate and explain your solution in person after the submission.
If such demonstration is needed, you will be contacted. If you do not defend/demonstrate in-person, no marks will be given, resulting in a mark of 0.
You must use modular design and modular programming
. Failure to use modular programming will result in a straight 0.
What you need to provide
Your assignment must contain the following components either in the documentation or as separate electronic files, where indicated:
1.
All assumptions made other than those stated in the question that you make about the problem. There will virtually always be assumptions you are implicitly making so think about this very carefully. Also, be careful that you do not put in unnecessary assumptions. (5%)
2.
Structure chart for your program. Make sure that your structure chart shows the parameter passing. (
5%
)
3.
Your algorithms written in a uniform fashion using a pseudocode or a similar style and adhering to the conventions required in the unit. Your algorithms should be presented at an appropriate level of detail sufficient to be easily implemented. Submit your high- level algorithm along with algorithms of your decompositions (refinements) as appropriate to the question. Algorithms that look like the code was written first and then word processed to look like an algorithm would receive no marks. (25%)
4.
A set of test data in tabular form with expected results and desk check results from your algorithm. Each test data must be justified – reason for selecting that data. No marks will be awarded unless justification for each test data is provided. (10%)
5.
Source code files (.c and .h) must be submitted and the source code must build (compile and link) to create an executable that operates correctly. Make sure you use the code style required in the unit. No marks awarded if the source code does not build and run. (50%)
6.
Results of applying your test data to your final program (tabular form), including screenshots of your program in operation
. You need to provide one screenshot per test case (5%)
2
Please submit the sections in order as shown in the assignment template in this document. Marks may
be lost otherwise.
3
Assignment Question
In this assignment, instead of asking the user to input the amount from the keyboard, this time, the program will read its data from a text file named coins.txt
. There can be any number of lines in the file. Each line is formatted like the example below:
Jane 30 cents in AU$
Joe 85 cents in EUR
Jane 25 cents in AU$
Jane 15 cents in US$
…
Names are one-word strings. The same name can be repeated in the data file, but the coin values/currencies can be different. If the name is the same, your program must assume it relates
to the same individual.
You are asked to write a program that reads the data from this type of files. For each individual, the program then must add up the coin amounts that belong to the same currency to obtain a total amount for that individual in that currency. It then needs to compute the change to be given for that individual for each of the currencies that that individual owns.
For example, the example input file above indicates that Jane has 30 + 25 = 55 cents in AU$ and 15 cents in US$. The program then needs to calculate how many coins (hereinafter referred to as
change) it should give to Jane for the amount it owns in AU$ and for the amount it owns in US$. The coins we have are the same as Assignment 1:
1) US$, which has four types of coins of 50, 25, 10 and 1 cents
2) AU$, which has four types of coins of 50, 20, 10 and 5 cents
3) Euro, which has four types of coins of 20, 10, 5 and 1 cents
The program should aim to give as much of the higher valued coins as possible (same as Assignment 1). Note that: -
The amounts in each line are between 1 to 95. However, when you sum up the all the lines of an individual, the total for that individual can exceed 95.
-
If the file contains a value that is not in that range, it should display a message saying that the data file has incorrect values and should display the line numbers that contain these incorrect values.
Once your program has read in the data from coins.txt
, your program will close coins.txt
first, and then show a console screen menu as illustrated below:
1. Enter name 2. Exit
The program will continue to show the menu and execute the menu options until "Exit" is selected by entering the value 2 at the menu prompt. When the user enters the value 1 at the menu prompt, your program will ask for a name. As an example, if the user enters the name Jane, the program 4
will output: Customer: Jane 55 cents in AU$ Change: 50 cents: 1 5 cents: 1 Jane 15 cents in US$ Change: 10 cents: 1 1 cents: 5
Change values of 0 should not be shown. If the user enters a non-existent name (e.g., Donald) at menu Option 1, which would not be in the array of records, your program will print:
Name: Donald
Not found
After the output is provided for menu Option 1, the menu is redisplayed.
Once the user enters 2 to exit, your program must write the coin, the currency and change data in .csv format for all names present in the file coins.txt to another file called change.csv
. After writing the data to the file your program must exit. In change.csv
, the data lines for Jane will look as follows, with each value separated by a comma and with the line terminated by newline: Jane, the change for 55 cents in AU$ is 1,0,0,1 Jane, the change for 15 cents in US$ is 0,0,1,5
So in the example output, Jane has -
55 cents in AU$: one 50 cent coin and one 5 cent coin. There are no 20 or 10 cent coins.
-
15 cents in US$: one 10 cent coin and five 1 cent coins. There are no 50 or 25 cent coins.
In the output data file change.csv
, if an individual’s name appears more than once inside coins.txt
,
their name should appear only once per currency inside change.csv
(with the accumulated coin amount and change for that currency).
You need to provide a test plan to fully test your algorithm and program, and provide an input data file, coins.txt
, that contains test data in the specified format for testing your program. Your solution (program and algorithm) should be modular in nature. Use a high cohesion and low coupling design.
5
Important Note:
For this problem, the principles of code reuse, low coupling and high cohesion,
covered in Week 6, are particularly important: A significant number of marks are allocated to these aspects of your design.
You should attempt to design your solution such that it consists of a relatively small number of modules (functions), but still meets the modular design best practice requirements of this unit.
In particular, strive to have one module that can be reused (called repeatedly) to solve the coin calculation problem. If you find that you have developed a large number of modules where each performs a similar task, or that you have a lot of instructions that are repeated in one module, then attempt to analyse your design to generalise the logic so that you have just one general version of the module.
Be mindful of the cohesion exhibited by each module. If you have a module that is doing more than one task (i.e. demonstrating low cohesion), then you should re-design it to have high cohesion.
Also, all the displays (i.e., printf) should happen in the main program, not in the individual
functions, except for the functions designed to ask the user to enter some input and those designed to print the final result.
Submit a structure chart and a high-level algorithm with suitable decompositions (refinement) of each step (low-level algorithm).
6
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
ASSEMBLY
It is preferable to pass Arrays by reference when calling subroutines.
True
False
arrow_forward
Query Board Python or Java(Preferred Python please) Thank you!
Programming challenge description:
There is a board (matrix). Every cell of the board contains one integer, which is 0 initially.The following operations can be applied to the Query Board:SetRow i x: change all values in the cells on row "i" to value "x".SetCol j x: change all values in the cells on column "j" to value "x".QueryRow i: output the sum of values on row "i".QueryCol j: output the sum of values on column "j".The board's dimensions are 256x256."i" and "j" are integers from 0 to 255."x" is an integer from 0 to 31.
Input:
Your program should read lines from standard input. Each line contains one of the above operations.
Output:
For each query, output the result of the query.
Test 1
Test InputDownload Test 1 Input
SetCol 32 20 SetRow 15 7 SetRow 16 31 QueryCol 32 SetCol 2 14 QueryRow 10
Expected OutputDownload Test 1 Input
5118 34
arrow_forward
1. Class
represents a dynamically resizable array-like data structure.
a. Stack
b. LinkedList
с. АrrayList
d. Array
2. A(n)
is a data structure with a fixed size and can be direct access its
elements.
a. Vector
b. ArrayList
c. LinkedList
d. Array
3. A(n)
is a data structure in which objects are inserted into and removed
from the same end.
a. LinkedList
b. ArrayList
c. Stack
d. Queue
4. A(n)
is a data structure in which objects are inserted into the end and
removed from the front.
a. LinkedList
b. ArrayList
c. Stack
d. Queue
arrow_forward
An array is a sequence of data items that are of the same type, that can be indexed, and that are stored contiguously. Typically,an array is called a data structure used to represent a large number of homogeneous values. The elements of an array are accessedby the use of subscripts. Subscripts start from zero and runs until less than the size of the array. Arrays of all types are possible,including multi-dimensional arrays. Strings are just arrays of characters terminated by a special character called the null character.A typical array declaration allocates memory starting from a base address. The array name is in effect a constant pointer to thismemory address.a) Write a complete C program that demonstrates the usage of character strings. The program should declare an emptycharacter array of specific size, and then ask user to enter a string to be stored in the array using a scanf function. Theprogram should finally display the string entered to the screen for checking if the…
arrow_forward
STRING-ARRAY
C program that scans a single char and prints out only the index/indexes of the character. If there are several matches, separate them by a single line.
INPUT- scans a single char
SAMPLE:Sample String: TheQuickBrownFoxJumpsOverTheLazyDogInput= TOutput= 0 26
arrow_forward
Material
Java/ C++/C- language
Personal Computer.
Instructions:
A. Matrix Addition
C. Matrix Transpose
1. The transpose of an m x n matrix A is n x m matrix AT.
2. Formed by interchanging rows into columns and vice versa.
3. (A¹)kj = Ajk
4. Let m input to enter the number of rows in the Matrix.
5.
Let n input to enter the number of columns in the Matrix.
6. Display the transpose matrix
7. Save the file TRANSPM
Questions:
1. What do you mean by an array?
2. Differentiate between for loop and while loop.
3. Define transpose of matrix? What will be the order of the matrix AT, if the order of the matrix A
is 4 x 4.
arrow_forward
Merge and quick sort Implementation – Directed Lab Work1. Complete the recursive algorithm for merge sort part in the given data file, ArraySortRecursive.java. 2. Complete the implementation of quick sort by implementing the method partition in thegiven data file, ArraySortRecursive.java.3. Save the file as ArraySortRecursiveYourlastname.java. 4. Save the test driver program as DriverYourlastname.java and run the program and verify.
arrow_forward
Material
Java/ C++/C- language
Personal Computer.
Instructions:
C. Matrix Transpose
1. The transpose of an m x n matrix A is n x m matrix AT.
2. Formed by interchanging rows into columns and vice versa.
3. (A¹)kj = Aj,k
4.
Let m input to enter the number of rows in the Matrix.
5. Let n input to enter the number of columns in the Matrix.
6.
Display the transpose matrix
7. Save the file TRANSPM
Questions:
1. What do you mean by an array?
2. Differentiate between for loop and while loop.
3. Define transpose of matrix? What will be the order of the matrix AT, if the order of the matrix A
is 4 x 4.
arrow_forward
Programming: C#(C sharp)
without using (array, while and furthermore advance loop)
Someone i know posted this here already but he got the wrong answer. I was hoping i can get the right one.
arrow_forward
A dynamic array is exactly as it sounds.
arrow_forward
Write every task in C++ programming language using arrays, array input/output and array operations.
Task 1:
Write a program that reads the characters from a text file. It counts total number of character and total number of vowels in the file.
arrow_forward
Data structure in C language:
Illustrate the following sorting methods by explaining their algorithm , mention their algorithm properties: time complexity and space complexity and various situations, stability, andweather it’s in place or not and the running time of the algorithm when the data array is:
1-Sorted (ascending)2-Sorted (descending)3-Not sorted
The algorithms:
1. Insertion2. Selection3. Bubble4. Shell5. Merge6. Heap7. Quick8. Radix
Please attach a clear photo
arrow_forward
C++ Progrmaing
Instructions
Redo Programming Exercise 6 of Chapter 8 using dynamic arrays. The instructions have been posted for your convenience.
The history teacher at your school needs help in grading a True/False test. The students’ IDs and test answers are stored in a file. The first entry in the file contains answers to the test in the form:
TFFTFFTTTTFFTFTFTFTT
Every other entry in the file is the student ID, followed by a blank, followed by the student’s responses. For example, the entry:
ABC54301 TFTFTFTT TFTFTFFTTFT
indicates that the student ID is ABC54301 and the answer to question 1 is True, the answer to question 2 is False, and so on. This student did not answer question 9 (note the empty space). The exam has 20 questions, and the class has more than 150 students. Each correct answer is awarded two points, each wrong answer gets one point deducted, and no answer gets zero points. Write a program that processes the test data. The output should be the student’s ID,…
arrow_forward
Course: Data Structure and Algorithms:
Language: Java
Please solve problem completely according to requirement, program should must be follow all the rules of menu base system which are required and write in question:
Attach the output's screentshots and explain every line with comments:
Whole code should be user defined inputs not fix:
Task : Address Book (List)• Your program will be a menu driven program. Address book will be implemented using the ArrayList.Description:
We want to build an address book that is capable of storing name, address & phone number of a person. Address book provides functionality in the form of a menu.
The feature list includes:• Add – to add a new person record• Delete – to delete an existing person record by name• Search – to search a person record by name· Exit – to exit from application
arrow_forward
Question 6 sm PYTHON please include comments to explain.
Create a recursive function that will recursively print a file list returned by os.listdir().
Full explain this question and text typing work only We should answer our question within 2 hours takes more time then we will reduce Rating Dont ignore this line
arrow_forward
JAVA CODE THANK YOU
2. Binary Search
by CodeChum Admin
Searching is one very important Computer Science task. When you have a list, searching is natural. You would want to search for an item in the list. If the list is not sorted, there is no way to it but do a linear search - check each element until the item is found or until there are no elements left to inspect.
But when the list is sorted, searching becomes way faster! Think of the dictionary; the actual printed dictionary. The words there are sorted from A-Z. And searching for what soliluquy means you should not start from A and turning the pages one by one until you reach the entries for the letter S.
What do you usually do? You open the dictionary right smack in the middle and see if you are in the letter S. If you are short and are brought to a letter before S, you try to estimate and open a page to the right of where you are currently. If you went beyond S, you try to open a page before the page you are currently…
arrow_forward
Data Structures are the programmatic way of storing data so that data can
be used efficiently. Almost every enterprise application uses various types of data
structures in one or the other way. There are various data structure techniques that can
be used for storing data systematically by applying good algorithms.
Design an algorithm that takes two arrays, and returns true if the arrays are
disjoint, i.e. have no elements in common. Use standard data structures and
algorithm in your solution without having to explain how they are
implemented. Write down your algorithm as pseudocode. You don't need to
write Java code but be precise so that a competent programmer should be able
to take your description and easily implement it. Your algorithm should take
Qn log m) time, where n is the size of the larger array and m is the size of the
smaller array. Since n2 m, this is the same as Q(n log m + m log m).
i.
ii.
Describe why it is a bad idea to implement a linked list version a queue which…
arrow_forward
Bishops on a binge def safe_squares_bishops(n, bishops):
A generalized n-by-n chessboard has been taken over by some bishops, each represented as a tuple (row, column) of the row and the column of the square the bishop stands on. Same as in the earlier version of this problem with rampaging rooks, the rows and columns are numbered from 0 to n - 1. Unlike a chess rook whose moves are axis-aligned, a chess bishop covers all squares that are on the same diagonal with that bishop arbitrarily far into any of the four diagonal compass directions. Given the board size n and the list of bishops on that board, count the number of safe squares that are not covered by any bishop.
To determine whether two squares (r1, c1) and (r2, c2) are reachable from each other in one diagonal move, use abs(r1-r2) == abs(c1-c2) to check whether the horizontal distance between those squares equals their vertical distance, which is both necessary and sufficient for the squares to lie on the same diagonal. This…
arrow_forward
How difficult is it to duplicate a collection of shared pointers into another array while using the C++ programming language? Create a list of the several approaches you may use to tackle the issue that has been presented to you. Is it the case that copying a shared pointer also copies the objects that it controls? Explain
arrow_forward
Rooks on a rampage
def safe_squares_rooks(n, rooks):
A generalized n-by-n chessboard has been invaded by a parliament of rooks, each rook represented as a two-tuple (row, column) of the row and the column of the square that the rook
is in. Since we are again computer programmers instead of chess players and other normal folks, our rows and columns are numbered from 0 to n - 1. A chess rook covers all squares that are in the same row or in the same column. Given the board size n and the list of rooks on that board, count the number of empty squares that are safe, that is, are not covered by any rook.
To achieve this in reasonable time and memory, you should count separately how many rows and columns on the board are safe from any rook. Because permuting the rows and columns does not change the answer to this question, you can imagine all these safe rows and columns to have been permuted to form an empty rectangle at the top left corner of the board. The area of that safe rectangle is…
arrow_forward
A dequeue is a list from which elements can be inserted or deleted at either end
a. Develop an array based implementation for dequeue.
b. Develop a pointer based implementation dequeue.
arrow_forward
programming: C#
without using array, while and loops we havent yet tackle those i just need the normal code.
arrow_forward
Hotel Reservation
- the python program should be applicable to real life situation (the program should describe a scenario or story)
- utilization of arrays will be a requirement: search algorithms and queue data structure
arrow_forward
C program
Andrew practices programming a lot and recently he came across the topic of pointers. He wondered if he could use pointers to print all prime numbers from an array? Could you help him by writing a C program which takes input in an array and uses a pointer to find and print all the prime numbers from the array.
Sample Run:
Input:
1 2 3 4 5
Output:
2 3 5
arrow_forward
Q/ Write a program in C# that lets the user to define the number of rows and columns. Then, the
program reads the matrix and finds the index of the even and odd numbers and display them with their
corresponding indices. Use adequate messages during the program execution.
arrow_forward
A dynamic array is what it sounds like.
arrow_forward
def delete task (..., ...):
I
param: task_collection (list) holds all tasks;
param:
an integer ID indexes each task (stored as a dictionary)
(str) - a string that is supposed to represent
an integer ID that indexes the task in the list
returns:
0 if the collection is empty.
-1 - if the provided parameter is not a string or if it is not a string
that contains a valid integer >=0 representing the task's position on the list.
Otherwise, returns the item (dict) that was removed from the
provided collection.
11 11 11
arrow_forward
Objectives
Java refresher (including file I/O)
Use recursion
Description
For this project, you get to write a maze solver. A maze is a two dimensional array of chars. Walls are represented as '#'s and ' ' are empty squares. The maze entrance is always in the first row, second column (and will always be an empty square). There will be zero or more exits along the outside perimeter. To be considered an exit, it must be reachable from the entrance. The entrance is not an exit.Here are some example mazes:mazeA
7 9
# # #####
# # # #
# # # ###
# # #
# ##### #
# #
#########
mazeB
7 12
# ##########
# # # #
# # # #### #
# # # #
# ##### ## #
# # #
############
mazeC
3 5
# # #
## ##
Requirements
Write a MazeSolver class in Java. This program needs to prompt the user for a maze filename and then explore the maze. Display how many exits were found and the positions (not indices) of the valid exits. Your program can display the…
arrow_forward
When working with enumeration types, such as the arithmetic
operators and the stream operators, is it possible to rapidly
access operators that are often used, such as the arithmetic
operators and the stream operators? Is it conceivable, for
instance, to overload these operators in order to arrive at a result
that is satisfactory? What are the arguments for and against
doing so?
arrow_forward
Computer Science
(PYTHON) Once a transaction completes, update the quantity on hand for each item sold to a customer in the dictionary:
Quantity = {101:2,102:5,103:8,104:2,105:8,106:4,107:6,108:3,109:2,110:10}
arrow_forward
Write every task in C++ programming language using arrays, array input/output and array operations.
Task 1:
Write a program that inputs the names of five cities from user in a string and stores/write them in a file city.txt
arrow_forward
File System: It is highly useful in file system handling where for example
the file allocation table contains a sequential list of locations where the files
is split up and stored on a disk. Remember that overtime it is hard for an
OS to find disk space to cover the entire file so it usually splits these up into
chunks across the physical hard drive and stores a sequential list of links
together as a linked list.
Write an algorithm for the above problem and analyse the efficiency of
the algorithm.
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
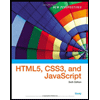
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
Related Questions
- ASSEMBLY It is preferable to pass Arrays by reference when calling subroutines. True Falsearrow_forwardQuery Board Python or Java(Preferred Python please) Thank you! Programming challenge description: There is a board (matrix). Every cell of the board contains one integer, which is 0 initially.The following operations can be applied to the Query Board:SetRow i x: change all values in the cells on row "i" to value "x".SetCol j x: change all values in the cells on column "j" to value "x".QueryRow i: output the sum of values on row "i".QueryCol j: output the sum of values on column "j".The board's dimensions are 256x256."i" and "j" are integers from 0 to 255."x" is an integer from 0 to 31. Input: Your program should read lines from standard input. Each line contains one of the above operations. Output: For each query, output the result of the query. Test 1 Test InputDownload Test 1 Input SetCol 32 20 SetRow 15 7 SetRow 16 31 QueryCol 32 SetCol 2 14 QueryRow 10 Expected OutputDownload Test 1 Input 5118 34arrow_forward1. Class represents a dynamically resizable array-like data structure. a. Stack b. LinkedList с. АrrayList d. Array 2. A(n) is a data structure with a fixed size and can be direct access its elements. a. Vector b. ArrayList c. LinkedList d. Array 3. A(n) is a data structure in which objects are inserted into and removed from the same end. a. LinkedList b. ArrayList c. Stack d. Queue 4. A(n) is a data structure in which objects are inserted into the end and removed from the front. a. LinkedList b. ArrayList c. Stack d. Queuearrow_forward
- An array is a sequence of data items that are of the same type, that can be indexed, and that are stored contiguously. Typically,an array is called a data structure used to represent a large number of homogeneous values. The elements of an array are accessedby the use of subscripts. Subscripts start from zero and runs until less than the size of the array. Arrays of all types are possible,including multi-dimensional arrays. Strings are just arrays of characters terminated by a special character called the null character.A typical array declaration allocates memory starting from a base address. The array name is in effect a constant pointer to thismemory address.a) Write a complete C program that demonstrates the usage of character strings. The program should declare an emptycharacter array of specific size, and then ask user to enter a string to be stored in the array using a scanf function. Theprogram should finally display the string entered to the screen for checking if the…arrow_forwardSTRING-ARRAY C program that scans a single char and prints out only the index/indexes of the character. If there are several matches, separate them by a single line. INPUT- scans a single char SAMPLE:Sample String: TheQuickBrownFoxJumpsOverTheLazyDogInput= TOutput= 0 26arrow_forwardMaterial Java/ C++/C- language Personal Computer. Instructions: A. Matrix Addition C. Matrix Transpose 1. The transpose of an m x n matrix A is n x m matrix AT. 2. Formed by interchanging rows into columns and vice versa. 3. (A¹)kj = Ajk 4. Let m input to enter the number of rows in the Matrix. 5. Let n input to enter the number of columns in the Matrix. 6. Display the transpose matrix 7. Save the file TRANSPM Questions: 1. What do you mean by an array? 2. Differentiate between for loop and while loop. 3. Define transpose of matrix? What will be the order of the matrix AT, if the order of the matrix A is 4 x 4.arrow_forward
- Merge and quick sort Implementation – Directed Lab Work1. Complete the recursive algorithm for merge sort part in the given data file, ArraySortRecursive.java. 2. Complete the implementation of quick sort by implementing the method partition in thegiven data file, ArraySortRecursive.java.3. Save the file as ArraySortRecursiveYourlastname.java. 4. Save the test driver program as DriverYourlastname.java and run the program and verify.arrow_forwardMaterial Java/ C++/C- language Personal Computer. Instructions: C. Matrix Transpose 1. The transpose of an m x n matrix A is n x m matrix AT. 2. Formed by interchanging rows into columns and vice versa. 3. (A¹)kj = Aj,k 4. Let m input to enter the number of rows in the Matrix. 5. Let n input to enter the number of columns in the Matrix. 6. Display the transpose matrix 7. Save the file TRANSPM Questions: 1. What do you mean by an array? 2. Differentiate between for loop and while loop. 3. Define transpose of matrix? What will be the order of the matrix AT, if the order of the matrix A is 4 x 4.arrow_forwardProgramming: C#(C sharp) without using (array, while and furthermore advance loop) Someone i know posted this here already but he got the wrong answer. I was hoping i can get the right one.arrow_forward
- A dynamic array is exactly as it sounds.arrow_forwardWrite every task in C++ programming language using arrays, array input/output and array operations. Task 1: Write a program that reads the characters from a text file. It counts total number of character and total number of vowels in the file.arrow_forwardData structure in C language: Illustrate the following sorting methods by explaining their algorithm , mention their algorithm properties: time complexity and space complexity and various situations, stability, andweather it’s in place or not and the running time of the algorithm when the data array is: 1-Sorted (ascending)2-Sorted (descending)3-Not sorted The algorithms: 1. Insertion2. Selection3. Bubble4. Shell5. Merge6. Heap7. Quick8. Radix Please attach a clear photoarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- New Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
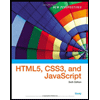
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning