final spring2022 answers
.pdf
keyboard_arrow_up
School
New York University *
*We aren’t endorsed by this school
Course
201
Subject
Computer Science
Date
May 13, 2024
Type
Pages
6
Uploaded by PhoenixY on coursehero.com
1 Computer Systems Organization CSCI-UA.0201-001 Spring 2022 Final Exam ANSWERS Write your answers on this exam paper. You can use the back of the sheets for scratch. 1.
(10 points) True/False. Please circle the correct response. a.
T A write-through cache doesn’t need a dirty bit associated with each cache entry. b.
F The output of a clocked latch (aka “D-latch”) changes only when the clock goes from high voltage to low voltage. c.
F The instruction “leaq 16(%rcx),%rdx” on the x64 processor could cause a cache miss if the contents of the memory location at address %rcx+16 is not in the cache. d.
T Assuming a two’s complement representation of signed integers, shifting a signed integer variable left by one bit in C could change the sign of the value of the variable. e.
F Assuming a two’s complement representation of signed integers, shifting a signed integer variable right by one bit in C could change the sign of the value of the variable. f.
T The instruction “addq -8(%rbp),%rax” will cause the write_enable line for the register file to be 1. g.
F A callee-saved register must be saved in a function even if the function is only reading the register (not writing it). h.
T Local variables in a function that are allocated on the stack will be at negative offsets from %rbp on the x64 processor. i.
F Formal parameters to a function that are passed on the stack (after the ones passed in registers) will be at negative offsets from %rbp on the x64 processor. j.
F In C, the operation “x & 0x8” will evaluate to 0 if bit 3 (the fourth lowest bit) of x is 0 and will evaluate to 1 if bit 3 of x is 1. 2.
(10 points: a is 4 points, the rest 2 points each) Fill in the blanks on this sheet. a.
Write the number E32A hex in binary: 1110 0011 0010 1010. b.
log 16T = 44 . c.
In order to access all bytes in a 64GB memory, an address must have at least 36 bits. d.
If a signed integer variable (using two’s complement) is represented by 42 bits, how many different negative numbers could that variable take on? Answer in multiples of K, M, G, or T. 2
42
/2 = 2
41
= 2T.
2 3.
(10 points) Write a C function whose prototype is int intlog(unsigned int num); and which returns the largest integer less than or equal to the log (base 2) of num
. For example, intlog(72)
should return 6, since 6 ≤ log 72 < 7. Answer: int intlog(unsigned int num) { int log = -1; // not really valid, assuming num > 0 while (num != 0) { num >>= 1; log++; } return log; }
4.
(15 points: a is 5 points, b is 10 points) Given the following definition of a QUEUE
struct type used for a queue, typedef struct { long head; long tail; char *buff[1000]; } QUEUE; where buff
is an array of strings, head
is the index of the first string in buff
, and tail
is the index of the last string in buff
, a.
Write a C function whose prototype is void insert_string(char *str, QUEUE *q); that takes a string (char pointer) and a pointer
to a queue and inserts the string into the queue’s buff
array after the last string already in the queue. Be sure to update the fields of the queue as necessary. You do not need to check if there is space available in buff
, you can just assume there is. This is a very short function. Answer: void insert_string(char *str, QUEUE *q) { q->tail++; q->buff[q->tail] = str; }
3 b.
Translate your C function into x86-64 assembly code for either Unix (e.g. MacOS or Linux) or Cygwin/Windows. An assembly reference sheet and the calling conventions for Unix and Windows are provided at the end of this exam. You can assume that the fields of a QUEUE
struct are contiguous (no space between them). Answer: _insert_string: pushq %rbp movq %rsp, %rbp # str is in %rdi # q is in %rsi # tail is at offset 8 # buff is at offset 16 incq 8(%rsi) # q->tail++; movq 8(%rsi),%rcx # %rcx holds q->tail movq %rdi,16(%rsi,%rcx,8) # q->buff[q->tail] = str; # each element is 8 bytes. popq %rbp retq 5.
(15 points: 5 points each question) a.
These questions relate to direct-mapped caches: i.
In a direct mapped cache whose capacity is K bytes (where K is a power of two), with one word per cache line, what is the relationship between the addresses of two words in memory that map to the same entry in the cache? Answer:
The addresses are a multiple of K apart. That’s because the cache index is computed as address mod size_of_cache. ii.
Suppose an address is 32 bits and there is a 1MB direct-mapped cache (where a word is 4 bytes). Not knowing anything else about the cache (e.g. size of the cache line), can you determine how many bits of an address is needed for the tag? If not, explain why not. If so, give the number of tag bits. Answer:
As indicated in the lecture, the tag is 30-log N bits, where N is the number of words in the cache, so in this case, the tag is 30-log(2
20
/4) = 30 – log(2
18
) = 30 – 18 = 12. Another way to look at it is that with a 1MB cache, to select a particular byte from the cache requires log(1M) = log(2
20
) = 20 bits, no matter how those bits are divided up into byte offset and word offset. The remaining 12 bits in the address must be the tag bits.
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
3. The table below represents five lines from a cache that uses fully associative mapping with a block size of 8. Identify the address of the shaded data, 0xE6, first in binary and then in hexadecimal. The tag numbers and word id bits are in binary, but the content of the cache (the data) is in hexadecimal.
Word id bits
Tag 000 001 010 011 100 101 110 111
------------------------------------------
1011010 10 65 BA 0F C4 19 6E C3
1100101 21 76 CB 80 D5 2A 7F B5
0011011 32 87 DC 91 E6 3B F0 A6
1100000 43 98 ED A2 F7 4C E1 97
1111100 54 9A FE B3 08 5D D2 88
arrow_forward
- A cache has been designed such that it has 1024 lines, with each line or block containing 8 words. Determine the line number, tag, and word position for the 20-bit address 3E9D216 using the direct mapping method.
arrow_forward
This chapter explains how to implement the four cache replacement policies.
arrow_forward
A 2-way set associative cache consists of four sets. Main memory contains 2K blocks of eight words each.
Show the main memory address format that allows us to map addresses from main memory to cache. Be sure to include the fields as well as their sizes.
Compute the hit ratio for a program that loops 6 times from locations 8 to 51 in main memory. You may leave the hit ratio in terms of a fraction.
Please show details how you obtain the result.
arrow_forward
A cache is set up with a block size of 32 words. There are 64 blocks in cache and set up to be 4-way set associative. You have byte address 0x8923. Show the word address, block address, tag, and index
Show each access being filled in with a note of hit or miss.
You are given word address and the access are: 0xff, 0x08, 0x22, 0x00, 0x39, 0xF3, 0x07, 0xc0.
arrow_forward
A 2-way set-associative cache consists of four sets. Main memory contains 2K blocks of 8 bytes each and byte addressing is used.Q.) Compute the hit ratio for a program that loops three times from addresses 0x8 to 0x33 in main memory. You may leave the hit ratio in terms of a fraction.
arrow_forward
Is there a way to find out which parts of a log entry a certain log processing function can read? The following code determines the typical number of cache misses per entry while using 64-byte cache blocks and no prefetching.
arrow_forward
A computer is using a fully associative cache and has 216 bytes of main memory (byte addressable) and a cache of 64 blocks, where each block contains 32 bytes. a. How many blocks of main memory are there? b. What will be the sizes of the tag, index, and byte offset fields? c. To which cache set will the memory address 0xF8C9(hexadecimal) map?
arrow_forward
In the event of an unsatisfied cache request, what should happen to the block being sent to the main memory from the write buffer?
arrow_forward
Q2: a cache memory consists of 512 blocks, and if the last word
in the block is 1111111. I the last Tag is 1111.
Then the total number of words in main memory is
Tags, and
The main memory consists of
blocks. The total number of bits in address fields i .
where number of bits for tag field is ....
and the remaining number of bits for word field i ..
arrow_forward
7. Show the final data and tags of the direct-mapped cache shown below for the given memory contents and
data access sequence. As shown, you have 8-bit addresses and 4 bytes of data in each block. You can
assume write-through and write-allocate write strategies on write hits and misses respectively.
Data Access Sequence
Address
Data
00 01 11 00
00 10 10 00
00 10 01 00
00 01 00 00
00 11 01 00
00 10 10 00
00 01 11 00
00 10 01 00
00 10 00 00
00 10 10 00
Op.
lw
lw
SW
lw
SW
lw
lw
SW
lw
lw
hits =
misses =
32
642
124
I
00
01
10
11
Direct-Mapped Cache
V Tag
Data
hit rate =
miss rate =
Memory
Address
00 00 10 00
00 00 11 00
00 01 00 00
00 01 01 00
00 01 10 00
00 01 11 00
00 10 00 00
00 10 01 00
00 10 10 00
00 10 11 00
00 11 00 00
00 11 01 00
Data
435
9
47
13
7586
86
43
48
673
4
972
1003
arrow_forward
In a microprocessor of 32 bit addresses, the tag length will change if we design a two-way set-associate cache versus a four-way set-associative cache, with the same number of cache lines in each organization, and the same number of data bytes per set.
a. True
b. False
arrow_forward
This function will be able to look at which fields in a log entry that it needs to. When you use 64-byte cache blocks and don't prefetch, the following code calculates the average number of cache misses for each entry in the cache.
arrow_forward
3. Caching
In this question, let's assume that we have a 16-bit system with a single level 6-way set associative
cache with 8 sets, and a cache block size of 64 bytes.
How many bits are needed for the setID and the tags? Draw the breakdown of the tag/index/byte-
in-block bits.
What is the total size of this cache?
For the following program, assume that an integer is 4 bytes.
int i; // Assume these variables are stored in the registers.
int a[65536]; // Assume that a = 0x1000
int b[65536]; // Assume that b = 0x8000000
for (i=0; i<1024; i++)
a[i* 8 ] = i;
for (i=0; i<1024; i++)
b[i * 8 ] = a[i * 8 ]++;
Let's asssume that the array is initialized to zero and that the variable i is already stored on the
register. What is my cache hit rate? Show your work.
arrow_forward
Clarify "unified cache" and "Hadley cache".
arrow_forward
In Figure 4.5, a flowchart of cache read operation is performed. Can you draw a cache write operation?
arrow_forward
The four cache replacement policies are explained in this chapter.
arrow_forward
A cache is set up with a block size of 32 words. There are 16 blocks in cache and set up to be direct map. You have byte address 0x8923. Show the word address, block address, tag, and index.
Show each access being filled in with a note of hit or miss.
You are given word address and the access are: 0xff, 0x08, 0x22, 0x00, 0x39, 0xF3, 0x07, 0xc0
arrow_forward
27. Why are the tag bits of a memory address important in a cache memory system?
A. The more tag bits there are, the higher the hit ratio.
B. The more tag bits there are, the lower the hit ratio.
C. The tag bits tell which block of main memory is occupying a cache line.
D. The tag bits are needed to tell whether a cache line has been modified or not.
E. The tag bits determine which level of cache is closest to the CPU.
arrow_forward
Describe the advantages and disadvantages of the two cache write policies.
arrow_forward
d. Find out tag-set-word bits for memory address: 24EA09CHfor two-way set associative (Assume cache
memory size 64 KB, number of bytes in each line is 8 bytes, and memory size is 64MB).
arrow_forward
Question 23
Some portion of cache system A represented below.
The system is byte-addressable and the block size is one word (4 bytes). The tag and line number are represented with a binary numbers. The contents of words in the block are represented with hexadecimal.
Tag
10 1000 0100 1001
10 1000 0100 1001
10 1000 0100 1001
10 1000 0100 1101
Line Number
0110 1101
0110 1110
0110 1111
B1 FF B8
A1 FF B8
B1 FF B8
A1 FF B8
B1 FF B8
0111 0000
1. What is the size of the main memory of this system?
2. What is the size of the cache memory of this system?
00
Word within block
2016 6116 C116 2116
01 10 11
3216 7216 C216 D216
4216 8216 4116 A216
E216 9216 5216 B216
3. If the CPU requests to read memory address A1 25 BA, what data does the CPU receive?
4. If the CPU requests to read memory address A1 35 C2, what data does the CPU receive?
5. If we access memory in the following order in cache system A:
A1 FF B8
how many cache misses would occur for the data request?
arrow_forward
Consider a 32-bit physical memory space and a 32 KiB 2-way associative cache with LRU
replacement. You are told the cache uses 5 bits for the offset field. Write in the number of bits
in the tag and index fields.
Tag length in bits =
Index length in bits =
arrow_forward
The phrases "unified cache" and "Hadley cache" should be defined.
arrow_forward
A CPU has 32-bit memory address and a 256 KB cache memory. The cache is organized as a 4-way set associative cache with cache block size of 16
bytes.
a. What is the number of sets in the cache?
b. What is the size (in bits) of the tag field per cache block?
c. What is the number and size of comparators required for tag matching?
d. How many address bits are required to find the byte offset within a cache block?
e. What is the total amount of extra memory (in bytes) required for the tag bits?
arrow_forward
Suppose a computer using fully associative cache has 224224 words of main memory and a cache of 512 blocks, where each cache block contains 16 words.
a. How many blocks of main memory are there?
b. What is the format of a memory address as seen by the cache, i.e., what are the sizes of the tag and offset fields?
c. To which cache block will the memory reference 1604181616041816 map?
Also...
Suppose a computer using set associative cache has 216216 words of main memory and a cache of 128 blocks, and each cache block contains 8 words.
a. If this cache is 2-way set associative, what is the format of a memory address as seen by the cache, that is, what are the sizes of the tag, set, and offset fields?
b. If this cache is 4-way set associative, what is the format of a memory address as seen by the cache?
arrow_forward
) Consider a direct-mapped cache with 128 blocks. The block size is 32 bytes. (03)
Find the number of tag bits, index bits, and offset bits in a 32-bit address.
Find the number of bits required to store all the valid and tag bits in the cache.
Given the following sequence of address references in decimal: 20000, 20004, 20008, 20016, 24108, 24112, 24116, 24120. Starting with an empty cache, show the index (line) and tag for each address and indicate whether a hit or a miss.
arrow_forward
6. For a direct mapped cache comprising 16 single word blocks answer the following
questions. Assume address and word sizes are both 32 bits and that the memory is
byte addressed (4 bytes per 32-bit word). Enter answers as numbers only.
How many index bits are there?
How many offset bits are there?
How many tag bits are there?
arrow_forward
Figurize the Fully associative cache (E = C/B) ?
arrow_forward
"Hadley cache" and "unified cache" need to be described in terms of what they mean.
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
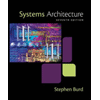
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
Related Questions
- 3. The table below represents five lines from a cache that uses fully associative mapping with a block size of 8. Identify the address of the shaded data, 0xE6, first in binary and then in hexadecimal. The tag numbers and word id bits are in binary, but the content of the cache (the data) is in hexadecimal. Word id bits Tag 000 001 010 011 100 101 110 111 ------------------------------------------ 1011010 10 65 BA 0F C4 19 6E C3 1100101 21 76 CB 80 D5 2A 7F B5 0011011 32 87 DC 91 E6 3B F0 A6 1100000 43 98 ED A2 F7 4C E1 97 1111100 54 9A FE B3 08 5D D2 88arrow_forward- A cache has been designed such that it has 1024 lines, with each line or block containing 8 words. Determine the line number, tag, and word position for the 20-bit address 3E9D216 using the direct mapping method.arrow_forwardThis chapter explains how to implement the four cache replacement policies.arrow_forward
- A 2-way set associative cache consists of four sets. Main memory contains 2K blocks of eight words each. Show the main memory address format that allows us to map addresses from main memory to cache. Be sure to include the fields as well as their sizes. Compute the hit ratio for a program that loops 6 times from locations 8 to 51 in main memory. You may leave the hit ratio in terms of a fraction. Please show details how you obtain the result.arrow_forwardA cache is set up with a block size of 32 words. There are 64 blocks in cache and set up to be 4-way set associative. You have byte address 0x8923. Show the word address, block address, tag, and index Show each access being filled in with a note of hit or miss. You are given word address and the access are: 0xff, 0x08, 0x22, 0x00, 0x39, 0xF3, 0x07, 0xc0.arrow_forwardA 2-way set-associative cache consists of four sets. Main memory contains 2K blocks of 8 bytes each and byte addressing is used.Q.) Compute the hit ratio for a program that loops three times from addresses 0x8 to 0x33 in main memory. You may leave the hit ratio in terms of a fraction.arrow_forward
- Is there a way to find out which parts of a log entry a certain log processing function can read? The following code determines the typical number of cache misses per entry while using 64-byte cache blocks and no prefetching.arrow_forwardA computer is using a fully associative cache and has 216 bytes of main memory (byte addressable) and a cache of 64 blocks, where each block contains 32 bytes. a. How many blocks of main memory are there? b. What will be the sizes of the tag, index, and byte offset fields? c. To which cache set will the memory address 0xF8C9(hexadecimal) map?arrow_forwardIn the event of an unsatisfied cache request, what should happen to the block being sent to the main memory from the write buffer?arrow_forward
- Q2: a cache memory consists of 512 blocks, and if the last word in the block is 1111111. I the last Tag is 1111. Then the total number of words in main memory is Tags, and The main memory consists of blocks. The total number of bits in address fields i . where number of bits for tag field is .... and the remaining number of bits for word field i ..arrow_forward7. Show the final data and tags of the direct-mapped cache shown below for the given memory contents and data access sequence. As shown, you have 8-bit addresses and 4 bytes of data in each block. You can assume write-through and write-allocate write strategies on write hits and misses respectively. Data Access Sequence Address Data 00 01 11 00 00 10 10 00 00 10 01 00 00 01 00 00 00 11 01 00 00 10 10 00 00 01 11 00 00 10 01 00 00 10 00 00 00 10 10 00 Op. lw lw SW lw SW lw lw SW lw lw hits = misses = 32 642 124 I 00 01 10 11 Direct-Mapped Cache V Tag Data hit rate = miss rate = Memory Address 00 00 10 00 00 00 11 00 00 01 00 00 00 01 01 00 00 01 10 00 00 01 11 00 00 10 00 00 00 10 01 00 00 10 10 00 00 10 11 00 00 11 00 00 00 11 01 00 Data 435 9 47 13 7586 86 43 48 673 4 972 1003arrow_forwardIn a microprocessor of 32 bit addresses, the tag length will change if we design a two-way set-associate cache versus a four-way set-associative cache, with the same number of cache lines in each organization, and the same number of data bytes per set. a. True b. Falsearrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
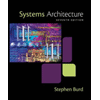
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning